Hilla 2.3 is out! This release includes a new AutoGrid component and authentication helpers for React.
🪄 AutoGrid: a fully-featured grid in one line of code
AutoGrid allows you to configure a Grid component to automatically fetch data from the backend. It supports pagination, sorting, and filtering out of the box.
<AutoGrid service={ProductService} model={ProductModel} />
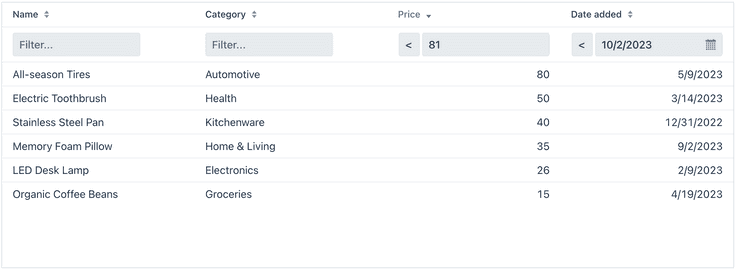
To work with AutoGrid, your backend service needs to implement the ListService
interface. If you are using Spring Data, you can also use the ListRepositoryService
for convenience:
@BrowserCallable
@AnonymousAllowed
public class ProductService extends ListRepositoryService<Product, Long, ProductRepository> {
}
Read more about AutoGrid in the documentation.
🛡️ React authentication helpers
Hilla 2.3 also adds new authentication helpers for React frontends.
AuthProvider
allows you to provide access to authentication information in your React components.
<AuthProvider> <RouterProvider router={router} /> </AuthProvider>
The protectRoutes
helper lets you define which routes require authentication. You can define either a list of roles or require the user to be logged in.
export const routes: RouteObject[] = protectRoutes([
{
path: "/products",
element: <ProductsView />,
handle: {
rolesAllowed: ["ADMIN"],
},
},
{
path: "/about",
element: <AboutView />,
handle: {
requiresLogin: true,
},
},
{
path: "/login",
element: <LoginView />,
},
]);
Finally, the useAuth
hook allows you to access the authentication information and login/logout functionality in your components.
export default function HelloWorldView() {
const {
state: { user },
} = useAuth();
return <h1>Hello {user ? user.name : "stranger"}!</h1>;
}
📦 How to upgrade
Upgrade your project by updating the Hilla version property in your pom.xml
file.
<properties>
<hilla.version>2.3.1</hilla.version>
<!-- ... -->
</properties>
If you don't already have a project, you can create one with the Hilla CLI:
npx @hilla/cli create my-app
📚 Full release notes
Read the full release notes on GitHub.